Java's default constructor tutorial
Java's default constructor allows developers to create instances of classes when no other constructors are explicitly defined.
The default constructor in Java takes no arguments -- it simply initializes reference types to null and primitive types to the binary equivalent of zero. That means instance variables are assigned as follows:
- Values for boolean are initialized to false.
- Values for floats and doubles are initialized to 0.0.
- Values for byte, short, int and long are set to 0.
- A char is set to Unicode's null character \u0000.
Why do we need default constructors in Java?
Here is an example of a class that implicitly provides a default constructor to the developer:
public class Score {
short wins;
long losses;
String playerName;
boolean userCheats;
char exitKey;
double moneyEarned;
}
The class in this example only defines properties. It contains no explicitly defined Java constructors.
When no explicitly defined constructors exist, Java's virtual machine makes object creation possible by providing a default constructor to developers who want to create instances of the class in their code.
Do not confuse the default constructor with the "no-args" constructor. If you write a zero-args constructor yourself, it is not the default constructor -- it replaces the default constructor.
Java default constructor example
Here is an example of the default constructor being used to create an instance of this class and print out the initial values of the class's properties.
void main() {
Score s = new Score(); // call to the Java class' default constructor
String output = "The default values are: %s %s %s %s %s %s";
System.out.printf(output,
s.wins, s.losses,
s.playerName, s.userCheats, s.moneyEarned, s.exitKey);
}
Default variable initialization
When this default constructor example code runs, it returns the following output:
The default values are: 0 0 null false 0.0
As you can see, the integer types are set to 0, decimal types are set to 0.0, Boolean values are set to false, and reference types are set to null. The null Unicode character of the char primitive type is not visible in the output.
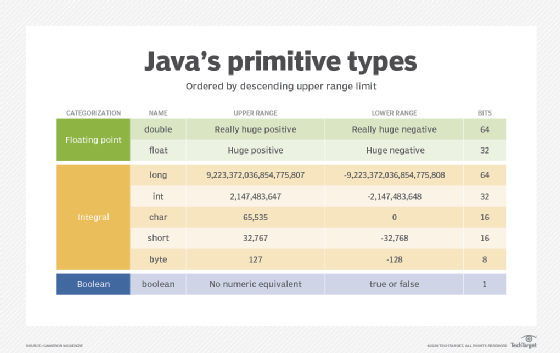
How to use Java's default constructor
Although the default constructor in Java is convenient and easy to use, developers must understand some important rules about its existence and implementation.
Follow these five guidelines when you include Java's default constructor in your code:
- A default constructor exists only when no other constructors exist in the class.
- The default constructor takes no arguments; its method signature is empty.
- You will never see the code that supports the default constructor; it is hidden from the developer.
- The default constructor includes an implicit call to the zero-argument constructor of its parent class.
- For more meaningful initializations of variables, you can provide a no-argument constructor of your own and override the default constructor.
When to use a default constructor
For simple classes where default initializations are acceptable, there's no need to create an exhaustive set of overloaded constructors.
However, as classes become more complicated and the initialization of instances becomes more nuanced, developers should provide a customized set of parameterized constructors to their users.
Cameron McKenzie has been a Java EE software engineer for 20 years. His current specialties include Agile development, DevOps and container-based technologies such as Docker, Swarm and Kubernetes.